Managing firmware download behavior
Deferring firmware download and installation
Firmware update can interrupt a device's regular functioning. For instance, image download requires the device to allocate buffers and communicate with the cloud, and the installation might require the device to reboot.
The device application can orchestrate the firmware update process by calling FOTA client's APIs to defer firmware download or installation and proceed with the download or install step when the device is ready.
This allows the device firmware application, which has contextual knowledge about the immediate needs of the device, to control the installation process, and provides a better user experience to device end-users by reducing interruptions to the device's regular functioning.
Resuming firmware download after an interruption
During a firmware download, an IoT device can lose power or connectivity for various reasons, including limited or intermittent availability of the battery or power source and unplanned power outages.
Instead of restarting the download after each interruption, the FOTA client lets the device resume the firmware download from the point at which the update process was interrupted.
Configuring defer update and resume download after interruption
To support the defer update or resume download after interruption features, set the MBED_CLOUD_CLIENT_FOTA_RESUME_SUPPORT
flag in your configuration file:
FOTA_RESUME_SUPPORT_RESUME
- Resumes download from the last successfully downloaded fragment. This is the default option.FOTA_RESUME_SUPPORT_RESTART
- Restarts download. This mode can be useful for constrained devices, and can help reduce code size.FOTA_RESUME_UNSUPPORTED
- Does not resume the update at all.
Note: The FOTA_RESUME_SUPPORT_RESUME
option is not available on devices with storage that does not support erasing; in other words, the FOTA client does not support resuming download from the last successfully downloaded fragment on devices that return a negative erase value in the fota_bd_get_erase_value
API. For more information about the fota_bd_get_erase_value
API, see fota_block_device.h
Integrating firmware update callbacks in your application
To control the update flow and defer and resume download, integrate the FOTA client callbacks below to your device application.
Note: If your application does not require an implementation of any special logic, the Device Management Client library provides a default implementation of the update callbacks. To enable the default FOTA callback implementation in Device Management Client, inject the FOTA_DEFAULT_APP_IFS
define into the application build.
-
fota_app_on_download_authorization()
- The FOTA download authorization callback.The client invokes this callback for the first time when the device receives the update manifest from the service, before downloading the image.
Your application can implement this callback and invoke one of the following APIs:
fota_app_authorize()
- Authorize request to download image. The download phase will proceed.fota_app_reject()
- Reject the FOTA request and discard the update. The update will not be re-prompted.fota_app_defer()
- Defer image download to a later phase. This aborts the current image download attempt, while preserving the update manifest. Download continues when the device application callsfota_app_resume()
. If the device reboots, the client calls thefota_app_on_download_authorization()
API to to request the device application's approval to continue the download.
The client expects the callback to return
0
(FOTA_STATUS_SUCCESS
) to acknowledge the authorization delivery. On constrained devices, the client expects the application to back up the current state and free resources (RAM), if necessary, before authorizing the download. -
fota_app_on_install_authorization()
- The FOTA update install authorization callback.The client invokes this callback for the first time when the device fully downloads the update candidate image, before installing the image.
The client expects the callback to call one of these APIs:
-
fota_app_authorize()
- Authorize FOTA to install the update candidate. The device may reboot or lose connectivity during candidate installation, depending on installer implementation. This phase is considered critical as power loss can brick the device. -
fota_app_reject()
- Reject the FOTA request to install and discard the update. The update will not be re-prompted. -
fota_app_defer()
- Defer the installation to a later phase. The client stops all operations related to image installation. Installation continues when the device applications callsfota_app_resume()
. -
fota_app_postpone_reboot()
- Postpone the device boot until the device application explicitly requests reboot.The client installs the update for the main component after the device reboots, without additional events or API calls after the installation.
If the device application supports component update, the client installs all components other than the main component when the device reboots, when the device next registers to Device Management or when the callback calls the
fota_app_resume()
API.
-
-
fota_app_on_complete()
- The FOTA update complete notification callback. The client notifies the application of completion or termination of the update process. The application may have postponed its main activities before authorizing an update. Therefore, the client notifies the application that the application can go back to its normal state.Note: The client does not call this callback if the system reboots as part of update installation.
-
fota_app_on_download_progress()
- Notifies the application about the firmware candidate download progress. The client calls this callback whenever the download progresses by approximately five percent. You can use the callback in the application to present the download progress visually.We recommend that you set this API during the development phase because it shows the download progress.
This diagram shows the FOTA callback calling sequence:
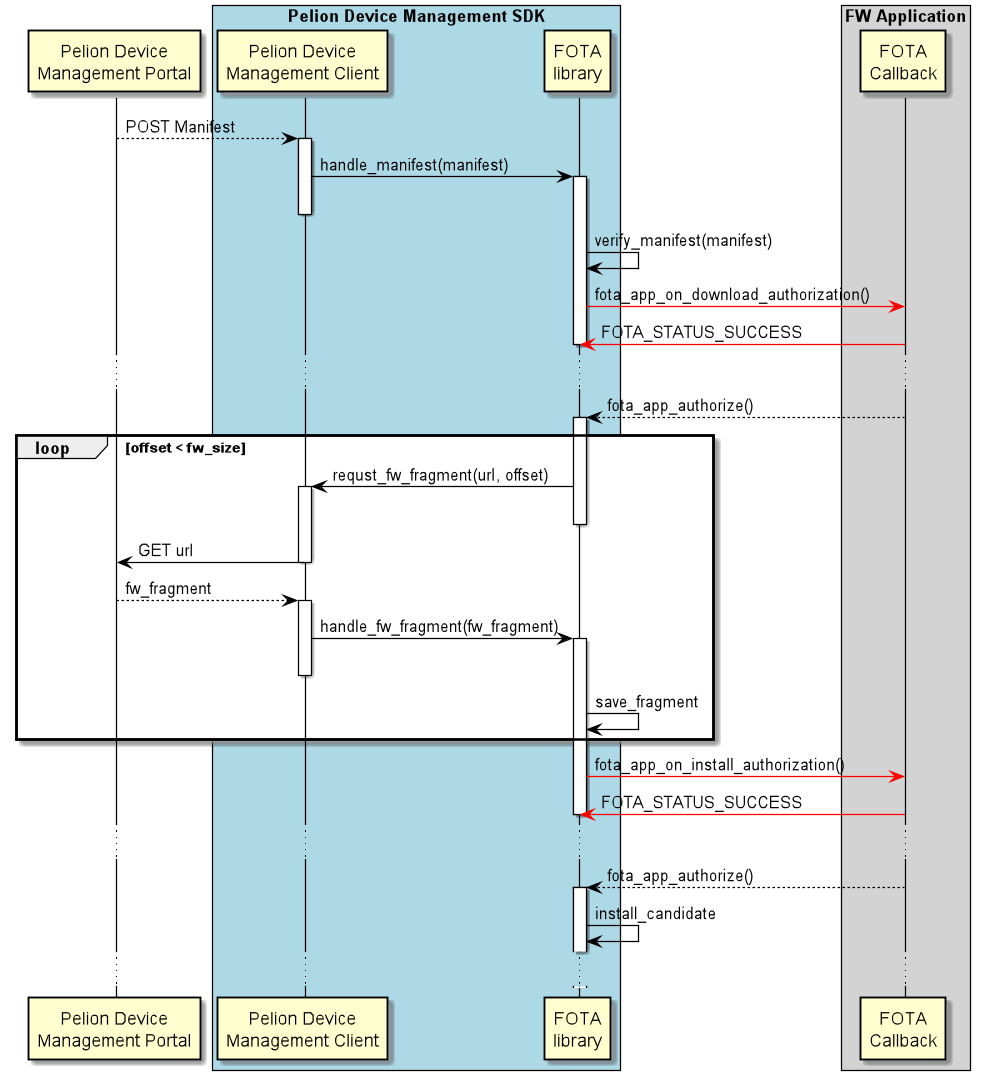
The red arrows show the application callback sequence in a good-path scenario in which the application authorizes all of the requests by calling fota_app_authorize()
. Note that the application callbacks call fota_app_authorize()
out of callback context to illustrate that the application can call these APIs from some event after handling ongoing business logic tasks.